Basic Programming - Three.js (WebGL)
Chapter 1 - Getting Started with Three.js
[iajsfiddle fiddle="ENUFy" height="500px" width="100%" show="result,js,html" skin="default"]
Step by Step Instructions
Configure JsFiddle as follows
- Framework & Extensions shows following Settings
- jQuery 1.9.1
- onLoad
- External Resource
- Add the url "http://threejs.org/build/three.min.js"
- Add the url "http://threejs.org/examples/js/controls/TrackballControls.js"
Create Skeleton Code
var renderer = createRenderer();
var scene = createScene();
var camera = createCamera();
var light = createLight();
var object3D = create3DObject();
scene.add(light);
scene.add(object3D);
renderer.render(scene,camera);
$("#container").append(renderer.domElement);
Create Renderer
var WIDTH=400;
var HEIGHT=400;
var createRenderer = function(){
var renderer = new THREE.WebGLRenderer();
renderer.setSize(WIDTH,HEIGHT);
return renderer;
}
Create Scene
var createScene = function(){
var scene = new THREE.Scene();
return scene;
}
Create Camera
var ASPECT = WIDTH/HEIGHT;
var ANGLE = 45;
var NEAR = 1;
var FAR = 1000;
var createCamera = function(){
var camera = new THREE.PerspectiveCamera(
ANGLE,ASPECT,NEAR,FAR);
//Pulling Camera back by 300 towards us
camera.position.z=300;
return camera;
}
Create Light
var createLight = function(){
var light = new THREE.PointLight(0xFFFFFF);
//Pulling light 100 points towards us
light.position.z=100;
return light;
}
Create Object3D - Skeleton
var create3DObject = function(){
var geometry = ..;
var material = ..;
var cubeMesh =
new THREE.Mesh(geometry,material);
return cubeMesh;
}
Create Object3D - Geometry & Material
var create3DObject = function(){
var geometry =
new THREE.CubeGeometry(30,30,30);
var material =
new THREE.MeshLambertMaterial(
{color:0xFF0000});
var cubeMesh =
new THREE.Mesh(geometry,material);
cubeMesh.position.x=0;
cubeMesh.position.y=0;
cubeMesh.position.z=0;
cubeMesh.rotation.x=45;
cubeMesh.rotation.y=45;
cubeMesh.rotation.z=45;
return cubeMesh;
}
Chapter 2 - Understanding WebGL Axis System
WebGL Axis System
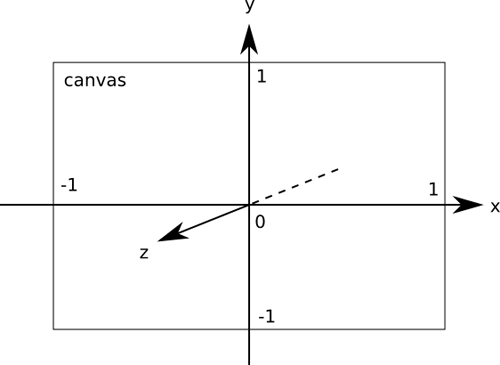
Three.js Program depicted Axis System
[iajsfiddle fiddle="YmJZM" height="500px" width="100%" show="result,js,html" skin="default"]
Create Custom Built 3D Axis
var createAxis=function(src,dst,colorHex,dashed){
var geom = new THREE.Geometry(),
mat;
if(dashed) {
mat = new THREE.LineDashedMaterial(
{
linewidth: 3,
color: colorHex,
dashSize: 3,
gapSize: 3
});
}
else {
mat = new THREE.LineBasicMaterial(
{
linewidth: 3,
color: colorHex
});
}
geom.vertices.push( src.clone() );
geom.vertices.push( dst.clone() );
// This one is SUPER important, otherwise
// dashed lines will appear as simple plain
// lines
geom.computeLineDistances();
var axis = new THREE.Line(
geom, mat, THREE.LinePieces );
return axis;
}
var createAxes = function(length) {
var axes = new THREE.Object3D();
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( length, 0, 0 ),
'red', false ) ); // +X
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( -length, 0, 0 ),
'red', true) ); // -X
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( 0, length, 0 ),
'blue', false ) ); // +Y
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( 0, -length, 0 ),
'blue', true ) ); // -Y
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( 0, 0, length ),
'green', false ) ); // +Z
axes.add( createAxis(
new THREE.Vector3( 0, 0, 0 ),
new THREE.Vector3( 0, 0, -length ),
'green', true ) ); // -Z
return axes;
}
Add Axis to Scene
scene.add(createAxes(100));
Chapter 3 - Playing with Animation
[iajsfiddle fiddle="qhbq9" height="500px" width="100%" show="result,js,html" skin="default"]
Instead of Rendering Directly use requestAnimationFrame
Replace following code snippet with
renderer.render(scene,camera);
this one
var render = function(){
renderer.render(scene,camera);
}
var animate = function(){
requestAnimationFrame(animate);
render();
}
animate();
Chapter 4 - Playing with Groups in Three.js
[iajsfiddle fiddle="WPgrF" height="500px" width="100%" show="result,js,html" skin="default"]
[iajsfiddle fiddle="pNrT7" height="500px" width="100%" show="result,js,html" skin="default"]
First Add the following Function to your Code
var createCube = function(
width,height,depth,color){
var cubeGeometry = new THREE.CubeGeometry(
width,height,depth);
var material =
new THREE.MeshLambertMaterial(
{
color:color
});
var cubeMesh = new THREE.Mesh(
cubeGeometry,material);
return cubeMesh;
};
Then create various Cube Objects
var cube1 = createCube(30,30,30,'red');
var cube2 = createCube(10,10,10,'yellow');
var cube3 = createCube(5,5,5,'green');
Then Organize the Cube Objects in Groups
var subGroup = new THREE.Object3D();
subGroup.add(cube2);
cube3.position.y=-40;
subGroup.add(cube3);
cube1.position.x=-40;
subGroup.position.x=30;
var group = new THREE.Object3D();
group.add(cube1);
group.add(subGroup);
Add the Group to the Scene
scene.add(group);
Finally modify the animate function to rotate cube, groups individually around different axis
var animate = function(){
requestAnimationFrame(animate);
cube1.rotation.x+= 0.05;
subGroup.rotation.z+= 0.05;
group.rotation.y+= 0.01;
render();
}
Chapter 5 - Playing with User Controls
Instructions
- Rotate - Left Mouse Click and Drag
- Pan - Middle Mouse/Scroll Wheel Click and Drag (OSX - 2 finger click and drag)
- Zoom - Scroll Wheel (OSX - 2 finger drag)
[iajsfiddle fiddle="jeq3W" height="500px" width="100%" show="result,js,html" skin="default"]
Add a TrackBall Control
var createControls = function(camera){
var controls = new
THREE.TrackballControls(camera);
controls.rotateSpeed = 1.0;
controls.zoomSpeed = 1.2;
controls.panSpeed = 0.8;
controls.noZoom = false;
controls.noPan = false;
controls.staticMoving = true;
controls.dynamicDampingFactor = 0.3;
controls.keys = [ 65, 83, 68 ];
return controls;
}
Render things when user changes the Camera using TrackBall Control
controls.addEventListener('change',render);
Also update the controls on each animationFrame
var animate = function(){
requestAnimationFrame(animate);
object3D.rotation.y+= 0.01
object3D.rotation.z+= 0.01
render();
controls.update();
}
Chapter 6 - Three.js Built in Helpers
Shows how to use inbuilt Grid Helper and Axis Helper
[iajsfiddle fiddle="tn9sm" height="500px" width="100%" show="result,js,html" skin="default"]
Use Ready made Axis code Provided by THREE.js
scene.add(new THREE.AxisHelper(100));
scene.add(new THREE.GridHelper(100,10));